tfListener can't find frames
Hello everyone,
in reference to a previous question, I wrote this simple urdf file:
<robot name="sonar_platform">
<!-- base link -->
<link name="base_link" />
<!-- sonar-ir fixed links -->
<link name="sonar0_link" />
<link name="sonar1_link" />
<joint name="sonar0_joint" type="fixed">
<parent link="base_link" />
<child link="sonar0_link" />
<origin xyz="1 1 0" rpy="0 0 0"/>
</joint>
<joint name="sonar1_joint" type="fixed">
<parent link="base_link" />
<child link="sonar1_link" />
<origin xyz="1 -1 0" rpy="0 0 1.57"/>
</joint>
</robot>
Then I use robot_state_publisher to publish the trasforms to tf using a very simple launch file:
<launch>
<param name="robot_description" command="cat $(find sonar_array)/platform_urdf.xml" />
<node name="robot_state_publisher" pkg="robot_state_publisher" type="state_publisher" />
</launch>
Finally, I implemented a tf listener based on the tutorial
#include <ros/ros.h>
#include <tf/transform_listener.h>
int main(int argc, char** argv){
ros::init(argc, argv, "sonar_array");
ros::NodeHandle node;
tf::TransformListener listener;
ros::Rate rate(10.0);
while (node.ok()){
tf::StampedTransform transform;
try{
listener.lookupTransform("/base_link", "/sonar0_link",
ros::Time(0), transform);
}
catch (tf::TransformException ex){
ROS_ERROR("%s",ex.what());
}
}
but when I try to launch it I get the following error:
[ERROR] [1312225092.766820278]: Frame id /base_link does not exist! When trying to transform between /sonar0_link and /base_link.
terminate called after throwing an instance of 'tf::LookupException'
what(): Frame id /sonar0_link does not exist! When trying to transform between /sonar0_link and /base_link.
Aborted
While, if I subscribe the /tf topic i can correctly see the transforms I defined:
transforms:
-
header:
seq: 0
stamp:
secs: 1312225269
nsecs: 108000004
frame_id: /base_link
child_frame_id: /sonar0_link
transform:
translation:
x: 1.0
y: 1.0
z: 0.0
rotation:
x: 0.0
y: 0.0
z: 0.0
w: 1.0
-
header:
seq: 0
stamp:
secs: 1312225269
nsecs: 108000004
frame_id: /base_link
child_frame_id: /sonar1_link
transform:
translation:
x: 1.0
y: -1.0
z: 0.0
rotation:
x: 0.0
y: 0.0
z: 0.706825181105
w: 0.707388269167
---
Any ideas on what's going on?
Comments
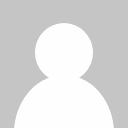
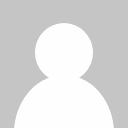
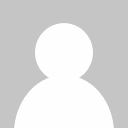